我写这个系列的目的,可能更倾向于自我总结,而不是教Flutter入门,所以可能有些东西是在我本人的理解下的一些用法与理解,不一定准确与适合所有开发者,如果有其他更好的想法,可以与我讨论,联系方式见关于
本项目基于macOS桌面程序,源码地址:https://github.com/NeverOvO/learningfoundation
-NeverOuO
Container属性
Container({
Key? key,
this.alignment,
this.padding,
this.color,
this.decoration,
this.foregroundDecoration,
double? width,
double? height,
BoxConstraints? constraints,
this.margin,
this.transform,
this.transformAlignment,
this.child,
this.clipBehavior = Clip.none,
}) : assert(margin == null || margin.isNonNegative),
assert(padding == null || padding.isNonNegative),
assert(decoration == null || decoration.debugAssertIsValid()),
assert(constraints == null || constraints.debugAssertIsValid()),
assert(clipBehavior != null),
assert(decoration != null || clipBehavior == Clip.none),
assert(color == null || decoration == null,
'Cannot provide both a color and a decoration\n'
'To provide both, use "decoration: BoxDecoration(color: color)".',
),
constraints =
(width != null || height != null)
? constraints?.tighten(width: width, height: height)
?? BoxConstraints.tightFor(width: width, height: height)
: constraints,
super(key: key);
alignment
用于确定Container内的child的对齐位置
一般用法:
Container(
child: Text("1233123"),
alignment: Alignment.centerLeft,
),
alignment属性:
Alignment(0.0, 1.0) , X取值[-1.0,1.0],Y取值[-1.0,1.0] , X确定左右方向,-1.0为最左,1.0为最右 Y确定上下方位,-1.0为最上,1.0为最下 快捷用法如下: Alignment.topLeft Alignment.topCenter Alignment.topRight Alignment.centerLeft Alignment.center Alignment.centerRight Alignment.bottomLeft Alignment.bottomCenter Alignment.bottomRight
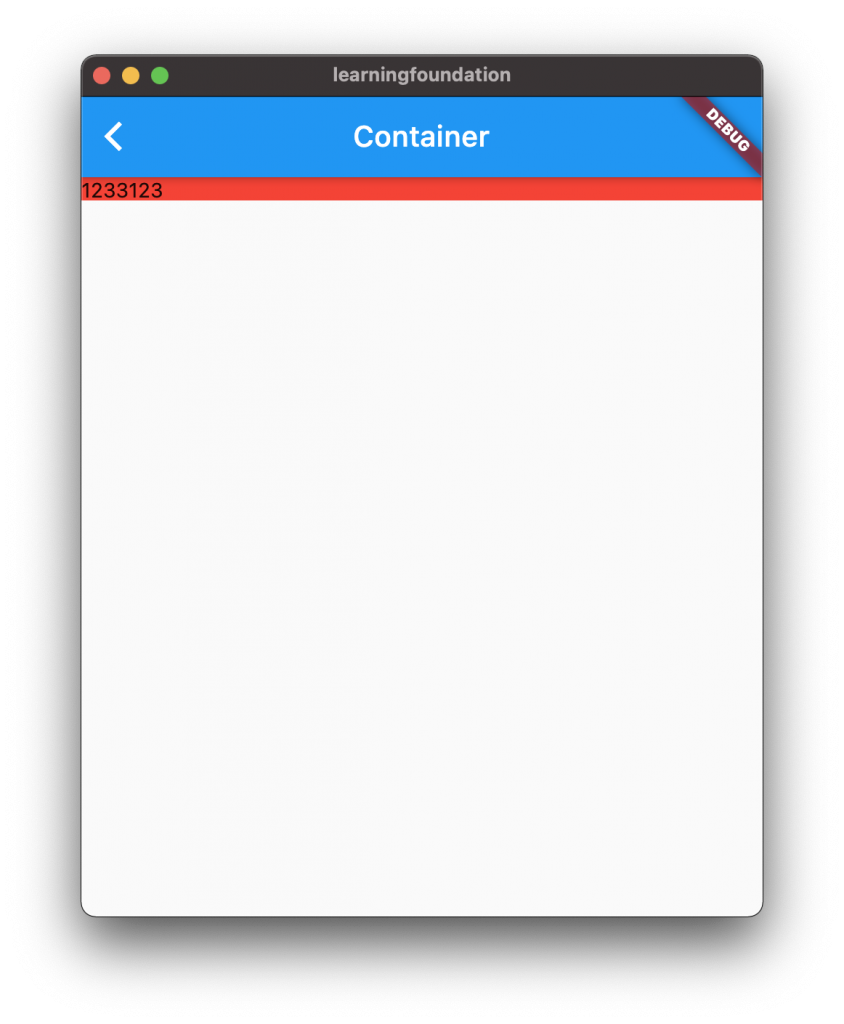
padding
用于确定Container内的child对于内边框的间隔距离
一般用法:
Container(
child: Text("1233123"),
color: Colors.red,
padding: EdgeInsets.fromLTRB(10, 20, 30, 40),
alignment: Alignment.centerLeft,
),
padding常用属性
EdgeInsets.fromLTRB(left, top, right, bottom),
EdgeInsets.only(),
EdgeInsets.all,
第一种用法最为常用,left,top,right,bottom,分别对应child对应内左边框,内上边框,内右边框,内下边框的距离;
only的用法为可选输入四边值,例如:EdgeInsets.only(left:10),等效于EdgeInsets.fromLTRB(10, 0, 0, 0);
all的用法为同时将四边距设为同一值,例如:EdgeInsets.all(10),等效于EdgeInsets.fromLTRB(10, 10, 10, 10);
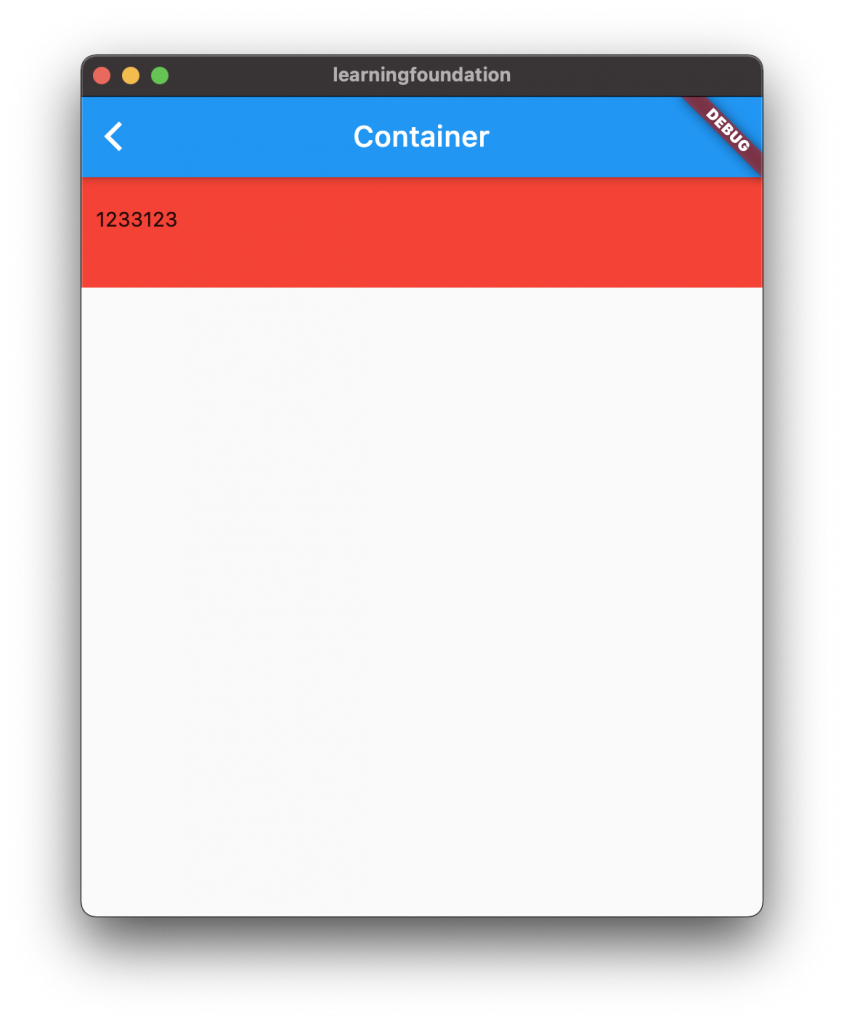
margin
用于确定Container对于程序窗口边框的距离,即外部距离
一般用法:
Container(
child: Text("1233123"),
color: Colors.red,
padding: EdgeInsets.fromLTRB(10, 20, 30, 40),
alignment: Alignment.centerLeft,
margin: EdgeInsets.fromLTRB(10, 20, 30, 40),
),
margin属性用法与padding相同,参考padding即可。
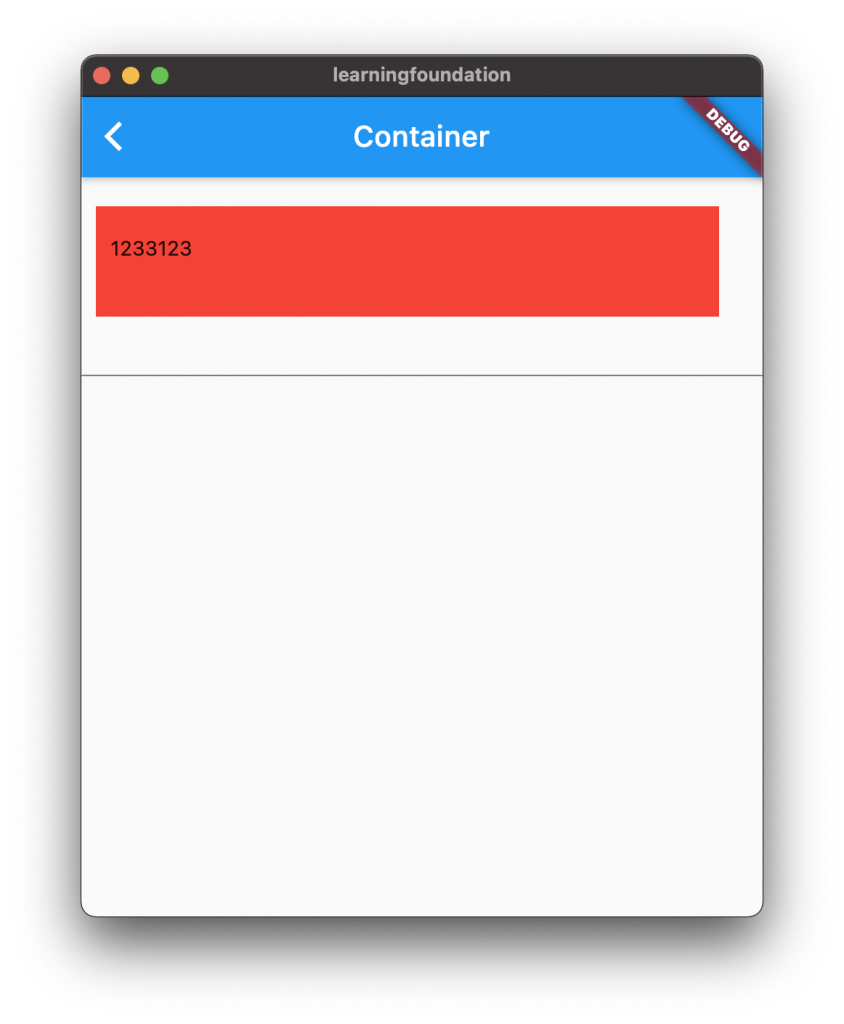
color
用于确定Container的背景颜色
decoration
这是Container的一个装饰外边缘或后边缘字段,可以深入许多内容,这边指罗列出我经常使用的几个属性
一般用法
Container(
child: Text("1233123"),
padding: EdgeInsets.fromLTRB(10, 20, 30, 40),
alignment: Alignment.centerLeft,
margin: EdgeInsets.fromLTRB(10, 20, 30, 40),
decoration: BoxDecoration(
//边框圆角设置
border: Border.all(width: 1, color: Color.fromRGBO(15, 159, 131, 1)),
borderRadius: BorderRadius.all(Radius.circular(3.0)),
image: DecorationImage(
image: AssetImage("images/con1.png"),
fit: BoxFit.cover,
),
boxShadow: [BoxShadow(blurRadius: 5, spreadRadius: 1, color: Colors.grey, offset:Offset(5,5)),],
),
),
拆分其中内容:
decoration: BoxDecoration(),个人常用用法;
若Container设置了decoration属性,则需要将color属性写到BoxDecoration下,否则会报错;
border: Border.all(width: 1, color: Color.fromRGBO(15, 159, 131, 1)),
设置外边缘的边框颜色与宽度
borderRadius: BorderRadius.all(Radius.circular(3.0)),
设置外边缘边框的圆角大小,可以使用only来设置,例如
borderRadius: BorderRadius.only(topRight:Radius.circular(30.0)),
此为仅设置了上右角的圆角大小,四个角皆可单独设置
image: DecorationImage(image: AssetImage("images/con1.png"),fit: BoxFit.cover,),
设置Container的背景图片,此属性的层级高于背景颜色层级,可以同时设置,但是有效时只会看到背景图片
boxShadow: [BoxShadow(blurRadius: 5, spreadRadius: 1, color: Colors.grey, offset:Offset(5,5)),],
设置Container的外阴影,
blurRadius:阴影范围,值越大这个颜色的边际越散、淡,
spreadRadius:阴影浓度,值越大范围内的颜色越深
color:阴影颜色
offset:阴影偏移量,Offset(5,5),即向右偏移5,向下偏移5
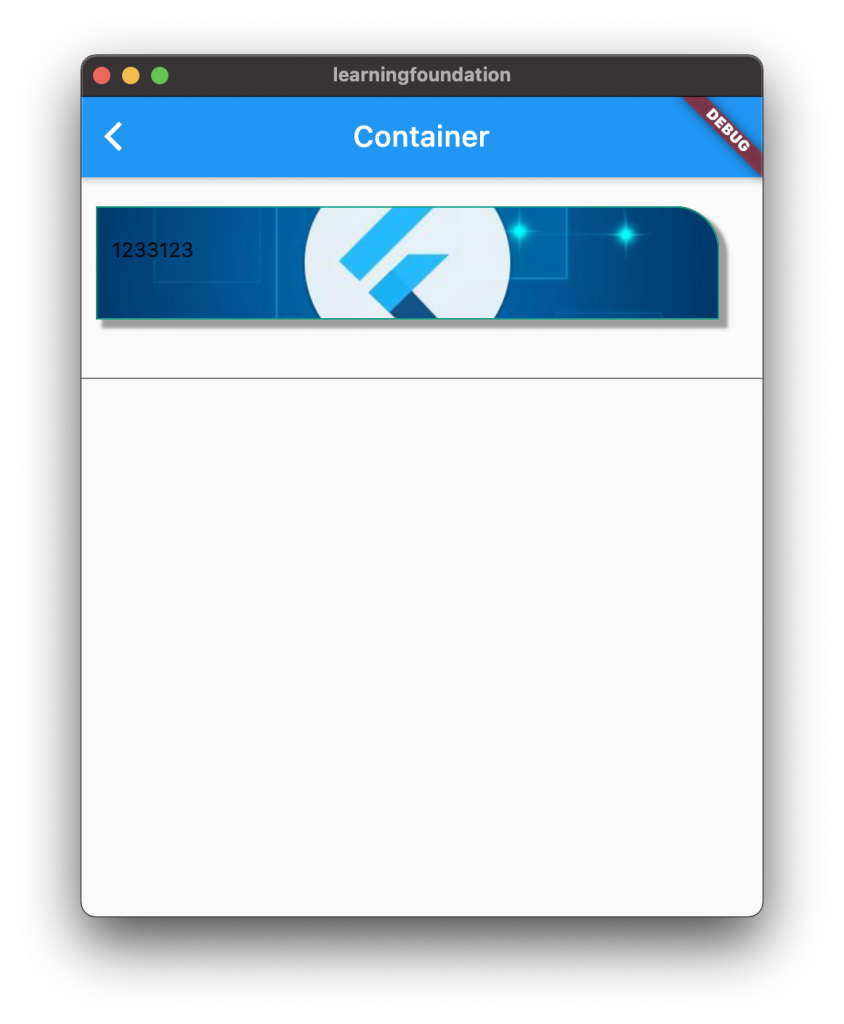
foregroundDecoration
可以理解为对内边框的装饰字段,参数可以参考decoration。
一般用法
foregroundDecoration: BoxDecoration(
color: Color.fromRGBO(12, 12, 12, 0.1),
border: Border.all(width: 3, color: Colors.red),
borderRadius: BorderRadius.only(bottomLeft:Radius.circular(30.0)),
),
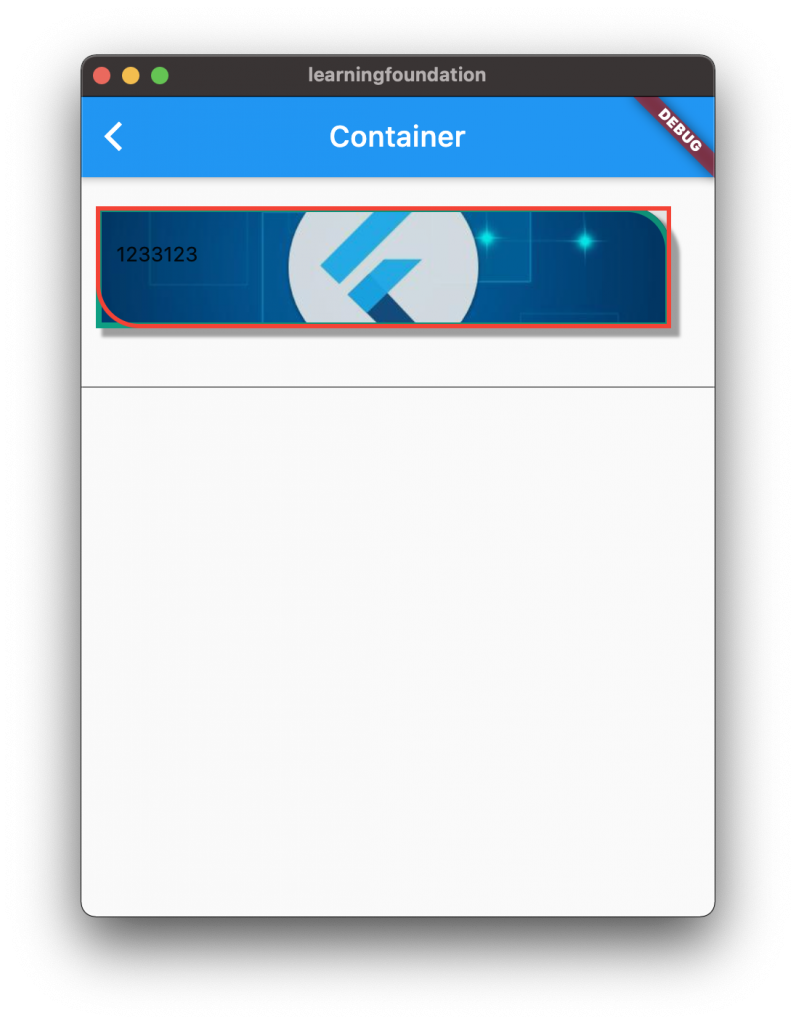
constraints
此参数为Container的约束性参数,可以限制组件的大小。
一般用法
Container(
child: Text("1233123"),
padding: EdgeInsets.fromLTRB(10, 20, 30, 40),
alignment: Alignment.centerLeft,
margin: EdgeInsets.fromLTRB(10, 20, 30, 40),
decoration: BoxDecoration(
//边框圆角设置
color: Colors.red,
border: Border.all(width: 4, color: Color.fromRGBO(15, 159, 131, 1)),
// borderRadius: BorderRadius.all(Radius.circular(30.0)),
borderRadius: BorderRadius.only(topRight:Radius.circular(30.0)),
image: DecorationImage(
image: AssetImage("images/con1.png"),
fit: BoxFit.cover,
),
boxShadow: [BoxShadow(blurRadius: 1, spreadRadius: 1, color: Colors.grey, offset:Offset(5,5)),],
),
foregroundDecoration: BoxDecoration(
color: Color.fromRGBO(12, 12, 12, 0.1),
border: Border.all(width: 3, color: Colors.red),
borderRadius: BorderRadius.only(bottomLeft:Radius.circular(30.0)),
),
constraints: BoxConstraints(
maxWidth: MediaQuery.of(context).size.width / 1.5,
),
),
属性
const BoxConstraints({
this.minWidth = 0.0,
this.maxWidth = double.infinity,
this.minHeight = 0.0,
this.maxHeight = double.infinity,
}) : assert(minWidth != null),
assert(maxWidth != null),
assert(minHeight != null),
assert(maxHeight != null);
约束窗口的最大最小宽高属性。
在不同屏幕适配时较为常用。
在桌面端上相比固定宽高,在窗口拉伸时显示限制效果更为合适。
transform
此参数为Container的变化参数,可以对widget进行平移、旋转、缩放等矩阵变换
一般用法
transform: Matrix4.skew(0.1,0.2),
即向X轴倾斜0.1,向Y轴倾斜0.2
同时还有skewX,skewY 即单独设置一轴
transform: Matrix4.rotationZ(0.1)
即向Z轴选择0.1
同时还有 rotationX,rotationY
Comments NOTHING